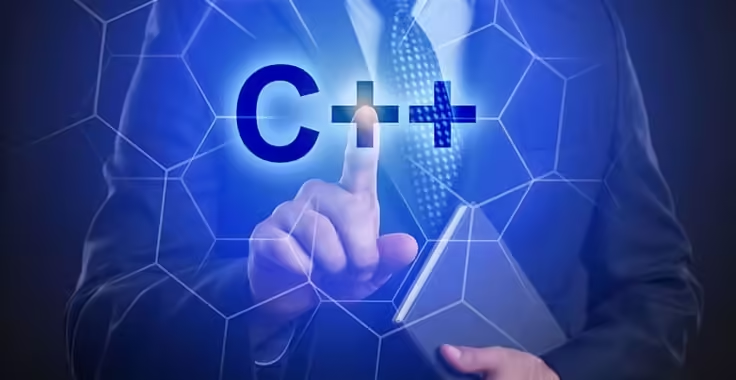
About Course
This course teaches you how to solve problems, both with and without code, with an emphasis on correctness, design, and style.
Register Now
What Will You Learn?
- This course teaches you how to solve problems, both with and without code, with an emphasis on correctness, design, and style.
Course Content
Introduction to computer science
-
Intro to Computer & Programs + Computational thinking (1)
-
Flowcharts & Pseudo code (2)
-
Binary representation + ASCII/Unicode (1)
-
practice / challenges session (1)
Basic Elements of C++
-
Quick look At C++ “Hello World!” Program
-
Basics of a C++ Program (Comments-Special Symbols-Reserved Words
-
Data Types + string Type and Variables (2)
-
Arithmetic Operators, Operator Precedence, and Expressions (1)
-
Type Conversion (Casting)
-
Variables, Assignment Statements, input/output (1)
-
practice / challenges session (1)
-
Increment and Decrement Operators
-
Creating a C++ Program + practice (1)
-
I/O Streams and Standard I/O Devices (1)
Control Structures I (Selection)
-
Control Structures, Relational Operators
-
Logical (Boolean) Operators and Logical Expressions (1)
-
Selection: if and if…else
-
One-Way Selection
-
Two-Way Selection
-
Compound (Block of) Statements
-
⦁ Multiple Selections: Nested if
-
⦁ Short-Circuit Evaluation
-
⦁ Conditional Operator (? :) (2)
-
⦁ switch Structures + practice (1)
Control Structures II (Repetition)
-
⦁ while Looping
-
⦁ do…while Looping
-
⦁ for Looping
-
⦁ break and continue Statements
-
⦁ Nested Control Structures (2)
-
⦁ practice / challenges session (1)
Functions
-
⦁ Predefined Functions
-
⦁ User-Defined Functions
-
⦁ Void Functions
-
⦁ Value-Returning Functions
-
⦁ Functions with Default Parameters
-
⦁ Reference Variables as Parameters
-
⦁ Value and Reference Parameters and Memory Allocation
-
⦁ Reference Parameters and Value-Returning Functions
-
⦁ Scope of an Identifier
-
⦁ Global Variables, Named Constants, and Side Effects
-
⦁ Static Variables
-
⦁ Function Overloading: An Introduction (4)
-
⦁ practice / challenges session (1)
⦁ Arrays
-
⦁ one dimensional array
-
⦁ two dimensional array
-
⦁ Multidimensional Arrays
-
⦁ Searching an Array for a Specific Item
-
⦁ C-Strings (Character Arrays) (2)
-
⦁ practice / challenges session (1)
-
⦁ Records (Struct) (1)
⦁ Pointers
-
⦁ Pointer Data Type and Pointer Variables
-
⦁ Address of Operator (&)
-
⦁ Dereferencing Operator (*)
-
⦁ Structs, and Pointer Variables
-
⦁ Initializing Pointer Variables
-
⦁ Dynamic Variables
-
⦁ Operations on Pointer Variables
-
⦁ Dynamic Arrays
-
⦁ Shallow versus Deep Copy and Pointers (4)
-
⦁ practice / challenges session (1)
⦁ Additional topics
-
⦁ Enumeration Type
-
⦁ Namespaces (1)
-
⦁ Additional string Operations (1)
-
⦁ Recursion / debugging skill (1)
-
⦁ practice / challenges session (1)
-
⦁ problem solving (1)
Bonus
-
⦁ 1 week workshop for final project
-
⦁ tricky interview question shared among all sessions